Sync Vs Async Programming In Python: Google Translation API
Sync and Async programming offer different approaches to optimize the performance of your applications. This guide uses the Google Cloud Translation API as an example.
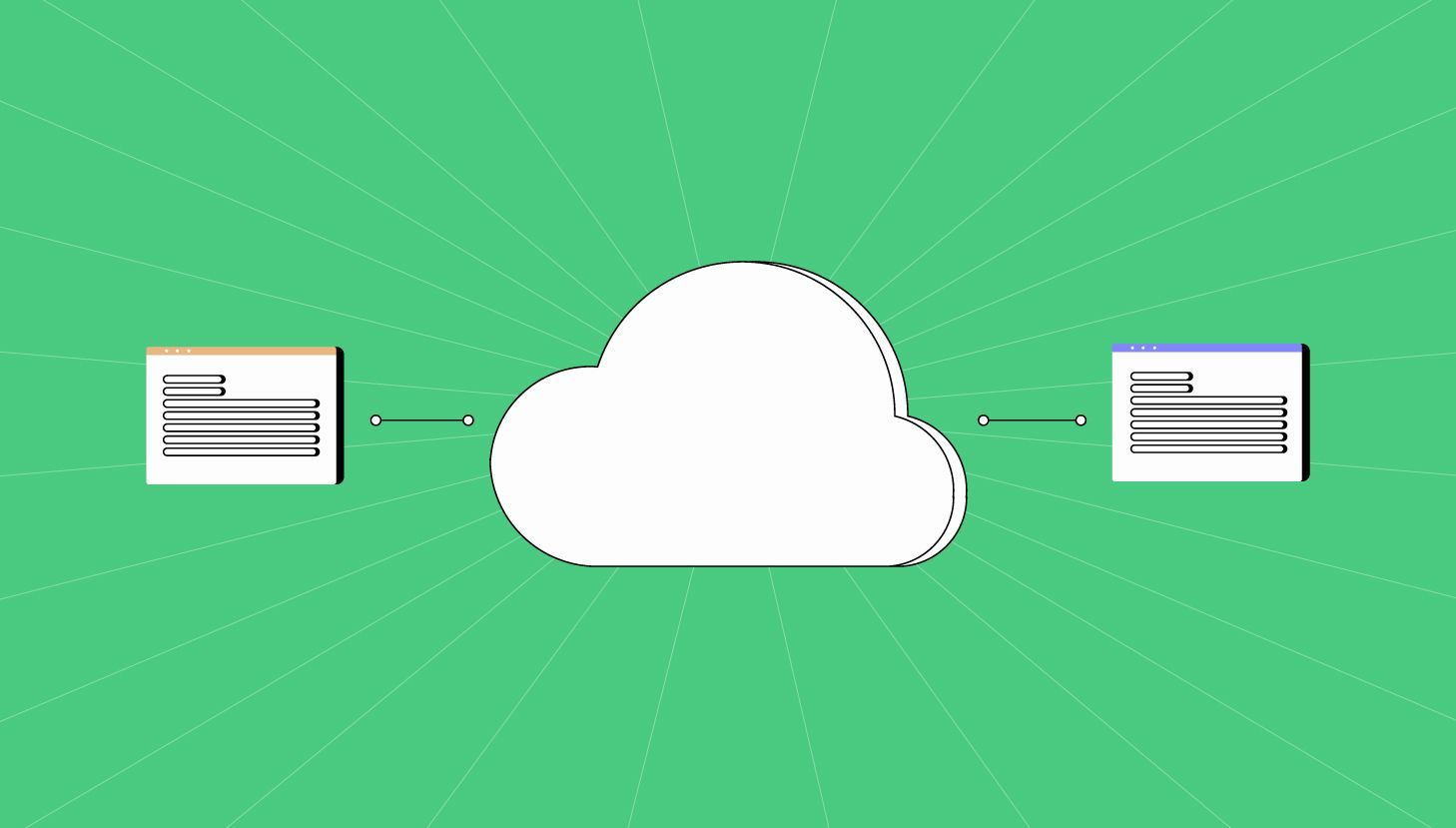
Introduction
In the world of software development, choosing the right programming approach is crucial to optimize performance and meet the requirements of your application. In Python, two popular methods for handling tasks are synchronous (Sync) and asynchronous (Async) programming. This guide will focus on comparing Sync and Async programming in Python using the Google Cloud Translation API as an example.
Synchronous (Sync) Programming
Synchronous programming in Python follows a sequential execution model. In this approach, each task is completed before moving on to the next one. Let's see how Sync programming can be used with the Google Cloud Translation API:
- Authentication and API Setup: Before using the Translation API, you need to set up authentication and create an API key. You can follow the steps provided by Google Cloud to set up your project and obtain the API key.
- Making Synchronous Translation Requests: With Sync programming, you make a translation request to the Google Cloud Translation API and wait for the response before proceeding. Here's an example using Python:
from google.cloud import translate_v2
translate = translate_v2.Client(target_language="en")
result = translate_client.translate('text you want to translate')
print(result['translatedText'])
In this example, the sync_translate_text
function sends a translation request to the API and waits for the response. The translated text is then returned and printed.
Asynchronous (Async) Programming
Asynchronous programming in Python allows for non-blocking execution of tasks. It leverages the use of coroutines, event loops, and callbacks to achieve concurrency. Here's how Async programming can be used with the Google Cloud Translation API:
- Authentication and API Setup: Same as with Sync programming, you need to set up authentication and obtain the API key for the Google Cloud Translation API.
- Making Asynchronous Translation Requests: With Async programming, you can submit multiple translation requests simultaneously and retrieve the results when they are ready. Here's an example using Python's
from google.cloud import translate_v3
texts_to_translate = ['comment allez-vous']
request = translate_v3.TranslateTextRequest(
contents=texts_to_translate,
target_language_code='en',
parent=f'projects/your-project-id'
)
response = await client.translate_text(request=request)
print([i.translated_text for i in response.translations])
In this example, the async_translate_text
function uses asyncio
to make an asynchronous translation request to the API. The loop.run_until_complete
method is used to wait for the translation to complete and retrieve the translated text.
Comparison and Choosing the Right Approach
When choosing between Sync and Async programming in Python, consider the following factors:
- Nature of the Application: Sync programming is suitable for applications that require sequential execution and immediate responses. Async programming is ideal for scenarios with a high volume of concurrent tasks and where responsiveness and scalability are crucial.
- Performance Requirements: If your application involves handling a large number of translation requests or requires parallel processing, Async programming can help improve overall performance by allowing non-blocking execution and concurrent processing.
- Development Team Expertise: Consider the experience and familiarity of your development team. If they have extensive experience with Sync programming and the application requirements do not demand Async features, sticking with Sync programming may be a more practical choice.
Conclusion
In Python, Sync and Async programming offer different approaches to handle tasks and optimize the performance of your applications. Sync programming provides simplicity and immediate responses, while Async programming enables parallel processing, scalability, and responsiveness.
By understanding the characteristics, benefits, and limitations of both approaches, you can make an informed decision based on your specific requirements. Whether you choose Sync or Async programming, Python provides a rich set of tools and libraries to support both styles of programming.
At Lykdat, we pride ourselves on providing cutting-edge solutions for gaining valuable insights from customer reviews through our Review Analysis solution. Recently, we incorporated the Google Cloud Translation API into our platform to offer seamless translation capabilities for our customers.